iOS Stringsdict Import / Export
Using the iOS Stringsdict file format to import/export pluralized content
Within the Localize dashboard we provide you with an ability to import and export phrases and translations in various file formats.
To see a general explanation of how importing and exporting works in Localize, click here.
UTF-8 File Format
Be sure that you are using the UTF-8 file format when working with the external files you are creating for importing purposes.
Importing Phrases
Before importing, make sure your file adheres to the file requirements below.
Navigate to the File Import / Export: Import tab in your dashboard.
- Drag-and-drop a file to import into the box, or select a file to import.
- Select IOS STRINGSDICT under File Format.
- Select the language you are importing.
- Specify the import type: Phrases or Glossary terms
- Click the Submit button.
- Wait for the file to be uploaded.
- Success! View the file details to see how many phrases were created, updated, etc.
After importing a source language file, Localize will show the phraseKey along with the phrase in the Localize dashboard.
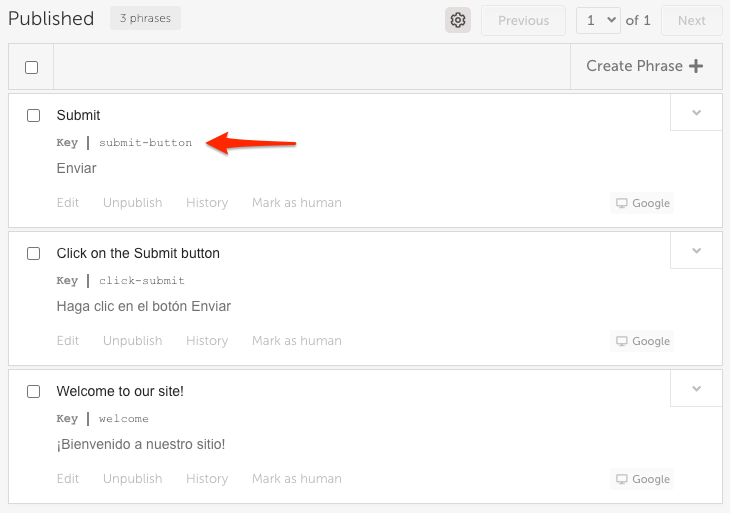
Exporting Phrases
Navigate to the File Import / Export: Export tab in your dashboard.
- Select IOS STRINGSDICT under File Format.
- Select a filter which will determine which phrases get exported:
a. Published translations
b. Machine translations
c. Human translations
d. No translations
e. Needs human translations - Select the language you are exporting.
- Specify the export type: Phrases or Glossary terms
- Click the Export button.
- Wait for your download to complete, then close the popup dialog.
Exporting Selected Phrases
Alternatively, you can filter exactly which phrases that you want to export on the Phrases and/or Glossary pages. Find out how...
File Requirements
iOS Stringsdict files contain a property list that's used to define plural rules for an iOS app. Localize has designed a very specific implementation of the Stringdict file format, defined below.
Each top-level key-dict
pair in the .stringsdict
file defines a plural rule for a specific phrase. Each phrase must have at least 1 numeric variable, and can have other variables as required.
Use the following sample file for guidance when creating your iOS Stringsdict file for importing into Localize.
Sample .stringsdict Source Language File
See the breakdown of this file below for details.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>person_has_numAnimals</key>
<dict>
<key>NSStringLocalizedFormatKey</key>
<string>%#@phrase@</string>
<key>phrase</key>
<dict>
<key>NSStringFormatSpecTypeKey</key>
<string>NSStringPluralRuleType</string>
<key>NSStringFormatValueTypeKey</key>
<string>i</string>
<key>one</key>
<string>%2$@ has 1 animal</string>
<key>two</key>
<string>%2$@ has 2 animals</string>
<key>other</key>
<string>%2$@ has %1$d animals</string>
</dict>
</dict>
<key>numPeople</key>
<dict>
<key>NSStringLocalizedFormatKey</key>
<string>%#@phrase@</string>
<key>phrase</key>
<dict>
<key>NSStringFormatSpecTypeKey</key>
<string>NSStringPluralRuleType</string>
<key>NSStringFormatValueTypeKey</key>
<string>i</string>
<key>one</key>
<string>There is one person</string>
<key>two</key>
<string>The are a pair of people</string>
<key>other</key>
<string>There are %1$d people</string>
</dict>
</dict>
</dict>
</plist>
This example contains 2 phrases, identified by the top-level keys
:
<key>person_has_numAnimals</key>
<dict>...</dict>
<key>numPeople</key>
<dict>...</dict>
Each phrase has a plural rule (NSStringLocalizedFormatKey). The plural rule determines the format string returned by the NSLocalizedString
macro. You supply a format string for each category of numbers the language defines. The <dict>
for each phrase has the following keys:
Plural Rule Key: NSStringLocalizedFormatKey
By definition, this is a format string that contains variables.
A variable is preceded by the %#@ characters and followed by the @ character.
Specifically for Localize, the format string contains only 1 variable, and that "variable" represents the entire phrase.
In our example phrases, we've named the variable phrase
.
[Variable] Key
Following the NSStringLocalizedFormatKey key, is a key that contains the variable above ("phrase" in our example).
<key>phrase</key>
It's <dict>
contains the following keys:
1. Language Rule Type Key: NSStringFormatSpecTypeKey
This key specifies the type of language rule, the value of which can only be NSStringPluralRuleType
, which indicates a language plural rule.
<dict>
<key>NSStringFormatSpecTypeKey</key>
<string>NSStringPluralRuleType</string>
...
</dict>
2. Format Specifier: NSStringFormatValueTypeKey
The value of this key contains a string format specifier for a number.
<dict>
...
<key>NSStringFormatValueTypeKey</key>
<string>i</string>
...
</dict>
3. Format String Keys
The remaining keys for the phrase dict contain the specific format stings to use for the number of "items" passed into the localizedStringWithFormat() method.
In our first example phrase, we define the format strings to use for the cases where the number of items is either zero, two or other.
For each format string, you will include the entire string for that case. In the strings you can specify where you want the variables to appear in the string by using the following notation:
- %1$d - this represents the value of the 1st parameter in the call to the NSLocalizedString macro, which is ALWAYS a number.
- %2$@ - this represents the value of the 2nd parameter in the call to the NSLocalizedString macro, in our case this will be a string.
- ...other parameters can be included as needed.
<dict>
...
<key>one</key>
<string>%2$@ has 1 animal</string>
<key>two</key>
<string>%2$@ has 2 animals</string>
<key>other</key>
<string>%2$@ has %1$d animals</string>
</dict>
Make sure you include an "other" case.
In general, if a plural type does not exist, such as “two”, “few”, “many”, or “zero”, the NSLocalizedString macro will fallback to the “other” case.
This is why it is required to have an other case for all phrases.
ViewController.swift
In your ViewController.swift file, you will be using the NSLocalizedString macro to retrieve the plural formats for the phrase, and then you will call the String.localizedStringWithFormat() function to get the specific instance for that phrase based on the number of items and other possible parameters passed into the function.
Below we show the output of a few examples with different input sent to the formatters.
Example #1:
Note that in this example, since there is no zero case, the format falls back to the other case.
let formatString = NSLocalizedString(“person_has_numAnimals", comment: "Any comments go here");
let resultString = String.localizedStringWithFormat(formatString, 0, "Sam");
print(resultString); // Result: "Sam has 0 animals"
Example #2:
let formatString = NSLocalizedString(“person_has_numAnimals", comment: "Any comments go here");
let resultString = String.localizedStringWithFormat(formatString, 0, "Kirk");
print(resultString); // Result: "Kirk has 1 animal"
Example #3:
let formatString = NSLocalizedString(“person_has_numAnimals", comment: "Any comments go here");
let resultString = String.localizedStringWithFormat(formatString, 10, "James");
print(resultString); // Result: "James has 10 animals"
Sample .stringsdict Target Language File
Sample plurals-es.stringsdict file in the target language of Spanish:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>person_has_numAnimals</key>
<dict>
<key>NSStringLocalizedFormatKey</key>
<string>%#@phrase@</string>
<key>phrase</key>
<dict>
<key>NSStringFormatSpecTypeKey</key>
<string>NSStringPluralRuleType</string>
<key>NSStringFormatValueTypeKey</key>
<string>i</string>
<key>one</key>
<string>%2$@ tiene 1 animal</string>
<key>two</key>
<string>%2$@ tiene 2 animales</string>
<key>other</key>
<string>%2$@ tiene %1$d animales</string>
</dict>
</dict>
<key>numPeople</key>
<dict>
<key>NSStringLocalizedFormatKey</key>
<string>%#@phrase@</string>
<key>phrase</key>
<dict>
<key>NSStringFormatSpecTypeKey</key>
<string>NSStringPluralRuleType</string>
<key>NSStringFormatValueTypeKey</key>
<string>i</string>
<key>one</key>
<string>Hay una persona</string>
<key>two</key>
<string>Son un par de personas</string>
<key>other</key>
<string>Existen %1$d personas</string>
</dict>
</dict>
</dict>
</plist>
Not available in Web projects
The iOS Stringsdict file format is not available in Web-based projects, because it is a format traditionally used in mobile app development.
Troubleshooting
If your import fails you can view the error here under Import History.
Updated over 4 years ago